Developed By:
Akshay Daga
The previous article explains serial communication using 8-bit data transfer. AVR microcontroller also supports serial data transfer with frame size of 5, 6, 7 and 9 data bits. The size of data frame can be adjusted according to application. For example, consider a system that needs to transmit only ASCII codes as data. In this case data frame size of 7-bits is sufficient because data length of ASCII code is equal to 7-bit. This will makes system more efficient by saving time of one clock period in each data frame transmission. This article explains serial transfer of data with different frame size.

The frame size of data can be selected by configuring the UCSZ (USART Character Size) bits. The Register UCSRC contains UCSZ [1:0] bits and UCSRB contains UCSZ2 bit. The following table shows the bit settings of UCSZ bits corresponding to different data frame size.
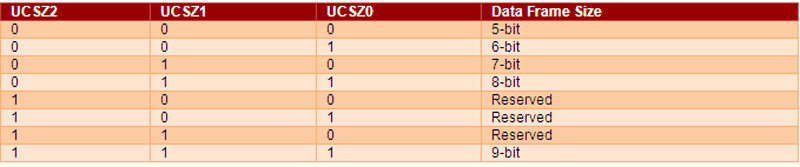
Fig. 2: Bit Setting of UCSZ for serial communication of different data frame size
Programming:
Case1: Frame size 5, 6 and 7 bits:
These three frame sizes can be selected by programming only UCSZ1 and UCSZ0 bits. There is no need to program UCSZ2 bit because default value of this bit is zero.
For example: UCSRC= (1<<UCSEL)|(1<<UCSZ0); // Asynchronous mode, frame size=6 bit,
// No parity, 1 stop bit.
Programming steps of sending and receiving data will remain same as used in 8-bit data transmission and reception. For more information, readers can refer the previous article on serial communication.
Test program for 6-bit reception and transmission:
A test program is written for 6-bit data communication between Microcontroller and PC. In this experiment the input is taken from the user through a keyboard. The corresponding data is sent to microcontroller via PC’s COM port. The microcontroller receives data and returns it again to PC’s COM port. The HyperTerminal is used to configure COM port to make it compatible for this experiment.
The following steps are used to configure COM ports:
1. Set the Baud rate = 9600 bps, data bit=6, Parity=None, Stop bit=1.

Fig. 3: Configure COM ports for serial communication
2. Tick the check box corresponding to Echo as shown in following picture to observe both transmitting and receiving data.

Output:
The following output is received when 1 2 3 4 5 6 A B C D E F G keys are pressed form keyboard.

Fig. 5: Output of Serial communication bewteen AVR and PC
As shown in above picture, the proper output is received when numeric keys are pressed but when alphabetic keys are pressed some symbol appears. This unexpected output is received because we are working with 6-bit data frame size. If 6-bit data frame is selected, two most significant bits are masked to zero while reading from UDR. The ASCII code of ‘1’ is 0x31 (0011 0001). If the two most significant bits are masked, 11 0001 remain which is also equal to 0x31. The size of ASCII codes for all numeric values is 6-bits so there will no problem in receiving and transmitting numeric values in 6-bit mode. But the ASCII value of ‘A’ is 0x41 (0100 0001). If two most significant bits are masked, 00 0001 remain which is equal to 0x01 so it will print some unusual symbol.
Case2: Frame size 9 bits:
9-bit data communication can be used in multi-processor communication. To select data frame of 9-bits, all UCSZ bits are set to 1(UCSZ [2:0] =7).
For example:
UCSRB=(1<<TXEN)|(1<<UCSZ2); // transmission on, UCSZ2 is set
UCSRC=(1<<URSEL)|(1<<UCSZ1)|(1<<UCSZ0);// Asynchronous mode, frame size=6 bit, // No parity, 1 stop bit.
1. 9-bit data transmission:
The 9-bit data has to be stored in buffer before transmitting. Since, data size of the UDR register is 8-bit only hence the ninth bit must be stored in TXB8 bit of the register UCSRB before lower byte is stored in UDR.
void usart_tx(unsigned int serial_data) //function transmission
{
while(!(UCSRA & (1<<UDRE))); // wait for empty UDR
UCSRB &= ~(1<<TXB8); //Copy 9-bit to TXB8
if(serial_data & 0x100)
UCSRB|=(1<<TXB8);
UDR=serial_data; // data put in UDR
}
2. 9-bit data reception:
The ninth bit must be read from RXB8 bit before reading lower byte. Status of FE (Frame Error), DOR (Data Over Run) and PE (Parity Error) must also be checked to verify the data.
unsigned int usart_rx(void) // function for reception
{
while(!(UCSRA & (1<<RXC))); // wait until reception is complete
if(UCSRA & ((1<<FE)|(1<<DOR)|(1<<PE))) //check FE,DOR and PE bits
return -1;
UCSRB|=(UCSRB>>1)&0x01; // filter 9-bit
return ((UCSRB<<8)|UDR); // return value
}
Circuit:

Code:
// Program for Serial communication (USART) with different frame size using AVR microcontroller
#define FREQ 12000000
#include<avr/io.h>
#include<util/delay.h>
#include<inttypes.h>
#define baud 9600
#define ubrr_value (((FREQ/16)/baud)-1)
void usart_init();
void usart_tx(unsigned char);
unsigned char usart_rx(void);
int main(void)
{
int serial_data;
usart_init();
while(1)
{
serial_data=usart_rx();
usart_tx(serial_data);
}
}
void usart_init() // USART initialization
{
UBRRH=(uint16_t)(ubrr_value>>8); // define UBRR value
UBRRL=(uint16_t) ubrr_value;
UCSRA=0x00;
UCSRB=(1<<TXEN)|(1<<RXEN); //enable both transmission and reception
UCSRC=(1<<URSEL)|(1<<UCSZ0); // Asynchronous mode,frame size=6 bit,No parity, 1 stop bit.
}
void usart_tx(unsigned char serial_data) // funtion for transmit bit
{
while(!(UCSRA & (1<<UDRE))); // wait for empty UDR
UDR=serial_data; // data store in UDR
}
unsigned char usart_rx(void)
{
while(!(UCSRA & (1<<RXC))); //wait until reception complete
return UDR; // receive value from UDR
}
Không có nhận xét nào:
Đăng nhận xét