
Fig. 1: Image showing AVR ATMega32 Microcontroller Board used in Light Tracking System
Electricity is the most required and important element of human life. We cannot imagine our day to day life without electricity. Electricity is generated using conventional (coal, diesel) and non conventional (water, wind, sunlight) energy sources. The recent and latest trend is to generate electricity from solar energy. The solar panel (made up of solar-photovoltaic cells) converts solar energy in to electrical energy. Several hundreds or thousands of solar panels to gather generates electrical energy in terms of Mega Watt (MW). For any small unit (single street light, solar light) single solar panel can used. Now to get maximum electrical energy from solar panel it is required that solar panel should always face towards sun. It should not be covered by any object (or obstacle) or should not come in shadow region. During day time sun moves from east to west. If solar panel is stationary it may be possible that it can be covered some time or may be in shadow region after a period. So there is a need of some mechanism that moves solar panel in the direction of sun. The arrangement should be made in such a way that solar panel automatically moves as it detects shadow region – that means less sunlight. In other way the solar panel should track sunlight (daylight).

Fig. 2: Image showing circuit connections of AVR ATMega32 Microcontroller Board used in Light Tracking System
The given project demonstrates how to move solar panel in the direction of light. It uses two LDRs as light sensor one for left and another for right and a stepper motor. The movement of stepper motor indicates movement of solar panel. When light is falling on both LDRs the motor is stationary. As light falling on any LDR increases it means other LDR is in shadow region or it is covered. So stepper motor moves in the direction of more light. Motor rotates till light falling on both LDR becomes same. That’s how motor rotates in either direction as light falling on any one LDR increases. The project uses AVR micro controller ATMega32 that will take controlling action to rotate stepper motor (solar panel) in the direction of maximum light.
Hardware & Software Connection
Hardware:
Circuit connection:
Major components are LDR-for sensing light, ATMega32 micro-controller, stepper motor driver UNL2003A and few LEDs – for indications.
· LDRs are connected between Vcc and Gnd with 10 K pots that provide bias to LDRs. Pots are used to set/change/adjust threshold level of falling light on LDR.
· The LDR junction outputs are fed to ADC0 and ADC1 input pins of AVR micro controller
· Four different colour LEDs are connected to PORTD pins with current limiting resistors. They are used to give different indications
· PORTC pins PC0 – PC3 are connected to input of UNL2003A.
· Four Outputs of UNL2003A are connected to four coils of stepper motor.
· Common coil terminal(s) of stepper motor is connected with Vcc supply
· A 16 MHz crystal with two 22 pf capacitors is connected to crystal input pins

Fig. 3: Image showing stepper motor controlled by the direction of incident light
Circuit operation:
· When light falling on both LDRs is same, the voltage at both ADC inputs are less than or more than threshold level. So motor is stop. This is indicated by RED led. This is possible in two cases. 1st when both LDR exposed to max light (less than threshold level) and 2nd when both LDR gets less light (more than threshold level)
· Now suppose light falling on LDR1 increases than LDR2. So input voltage at ADC0 increases threshold level. But at ADC1 it is still below threshold level. (this means LDR2 may be in shadow region) So motor rotates in clockwise direction to rotate panel in the direction of LDR1 to get max light on both LDRs. This is indicated by GREEN LED
· Motor rotates till both ADC inputs become less than threshold level
· Similarly when light falling on LDR2 increases, motor rotates in anticlockwise direction. This is indicated by YELLOW LED
· Micro-controller ATMega32 reads analog voltage values of LDR1 and LDR2 after every 500 ms. This is indicated by blinking of BLUE LED. So falling light on both LDR is sensed at a rate of 2 samples / second.
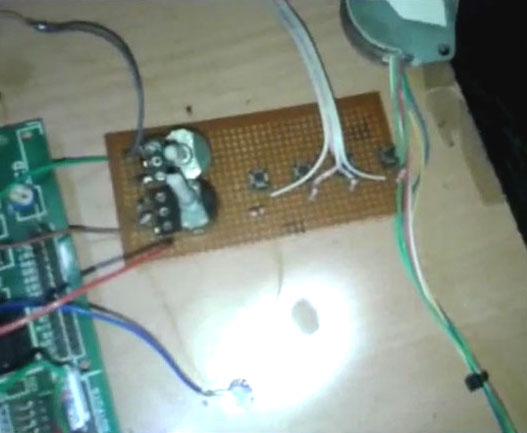
Fig. 4: Image showing Blue LED glowing as indication of light intensity sensed by LDR sensors
Software:
The software program embedded into AVR micro controller is responsible for complete functionality. It performs following tasks
1. Reads analog voltage values and converts it in to digital equivalent
2. It converts this digital value into equivalent decimal value
3. Compare these decimal values with threshold levels to make decision to rotate motor
4. Give pulses to stepper motor to rotate it clockwise or anticlockwise
5. Give different indication on LEDs
The program is written in C language. It is compiled using AVR GCC compiler and simulated using AVR simulator for ATMega32 micro-controller. Both AVR GCC compiler and AVR simulator are available builtin in AVR studio (V4) software tool. When program is compiled successfully it generates HEX file. This file can be loaded into target device ATMege32 using any suitable programmer (like ponyprog). Here is the complete program code with necessary comments.
.
Circuit:
Code:
#include <avr/io.h> // include necessary header files
#include <util/delay.h>
unsigned int c=0; // initialize count
void adcinitliz() // initialize built in ADC
{
ADMUX = (1<<REFS0);
ADCSRA =(1<<ADPS2) | (1<<ADPS1) | (0<<ADPS0);
}
unsigned int convert() // convert 10 bit HEX value into
{ // decimal equivalent
unsigned int tmp1,tmp2,tmp3,t;
tmp1 = (ADCL & 0x0F);
tmp2 = ADCL >> 4;
tmp3 = (ADCH & 0x0F);
tmp2 = tmp2*16;
tmp3 = tmp3*256;
t = tmp1+tmp2+tmp3; // and return decimal value
return t;
}
void main()
{
unsigned int sen1_value,sen2_value,i;
DDRC=0x0F; // PORTC as output
PORTC=0x00;
DDRD=0x0F; // PORTD as output
PORTD=0x00;
adcinitliz(); // initialize ADC
while(1)
{
PORTD = 0x08; // indication on BLUE LED
if(c<3) c++; // increase count every time
if(c==1) ADMUX=0x00; // for count=1 select 1st ADC channel
else if(c==2) ADMUX=0x01; // or select 2nd ADC channel
ADCSRA = (1<<ADEN) | (1<<ADSC); // start ADC
while(!(ADCSRA & (1<<ADIF))); // wait till end of conversion
ADCSRA = (1<<ADIF);
if(c==1) sen1_value = convert(); // get the value of LDR1
else if(c==2) {sen2_value = convert();c=0;} // get the value of LDR2
// for both values less than threshold value, stop motor
if(sen1_value<=512 & sen2_value<=512) {PORTC = 0x00;PORTD |= 0x01;}
// for both values more than threshold value, stop motor
else if (sen1_value>512 & sen2_value>512) {PORTC=0x00;PORTD |= 0x01;}
// if LDR1 value is more than threshold
else if (sen1_value>512 & sen2_value<=512)
{
for(i=0;i<5;i++) // give pulse sequence to rotate motor
{ // clockwise
PORTD |= 0x02; // indication on GREEN LED
PORTC=0x01;
_delay_ms(100);
PORTC=0x02;
_delay_ms(100);
PORTD &= 0xFD;
PORTC=0x04;
_delay_ms(100);
PORTC=0x08;
_delay_ms(100);
}
}
// if LDR2 value is more than threshold
else if(sen1_value<=512 & sen2_value>512)
{
for(i=0;i<5;i++) // give pulse sequence to rotate motor
{ // anticlockwise
PORTD |= 0x04; // indication on YELLOW LED
PORTC=0x08;
_delay_ms(100);
PORTC=0x04;
_delay_ms(100);
PORTD &= 0xFB;
PORTC=0x02;
_delay_ms(100);
PORTC=0x01;
_delay_ms(100);
}
}
_delay_ms(250); // give delay for 250 ms
PORTD &= 0xF7; // BLUE LED off
_delay_ms(250); // again give delay of 250 ms
}
}
Không có nhận xét nào:
Đăng nhận xét