This project is just a smart version of any keypad lock. What’s smart about it is that it can detect whether it is needed by the user or not and accordingly switches itself to take a sleep. Making a microcontroller to sleep reduces power consumption as well as increases its usage span.
Many of you must be wondering that would it be secure enough to make a lock go to sleep, isn’t it. Don’t worry, when a microcontroller goes off to sleep it puts a hold on what it was doing before sleeping. In my project I have made it to sleep only when the user has finished entering the password stuff and microcontroller has done its entire job.
This was really necessary because I didn’t find it logical to make the microcontroller keep active all the time even when it’s not needed.
So, after entering the sleep mode it can be woken up by any interrupt source. Here I have used 4 diodes in such a way that when any of the keypad buttons is pressed they generate a low level pulse at the external interrupt INT0. INT0 is set to call its ISR routine function when such a thing happens. In short these diodes are working as an OR gate to detect any key pressed.
After waking up, the microcontroller resumes from the position where it went to sleep, thereby avoiding any risk of data mishandling.
For further references you can see the coding part as well.
The Keypad is connected to PORTC and the LCD works on 4-bit mode with PORTB.
I have used a self-made header file for the keypad interfacing which you can find in this folder itself.
A great thing about this lock is that it can remember your changed password. Now the question is how to change the password? It can be done when the safe is open; you just need to enter the new password that you want. Note that this password won’t be hidden, as it was at the unlocking time.
In other words at the time of unlocking, the LCD screen displays “*” for each time user presses a button. This is done to secure the password. But at the time of renewing it, LCD displays the same character pressed on the keypad just to ensure user about the password. Once the password has been changed everything becomes hidden again.
One more feature about this lock that would excite some of you is that you can even erase and rewrite your password. For erasing purposes, “<-” this button needs to be pressed.
In my project I have used an LED as an indication about the lock status. When LED lights up it means unlocked status and when LED switches off it indicates the locked status.
In the coding part variable “i” plays an important role in deciding whether the microcontroller will go to sleep or not. A while loop runs till i<15000. “i” is incremented every time by one when no button is pressed but as soon as any button is pressed its value reaches to 0 and then again the whole process works accordingly.
Requirements
• Keypad 4X4
• Diode (1N4007)-4
• AtMega16
• LCD (16X2)
• Locking Mechanism (optional)
Code:
Circuit:
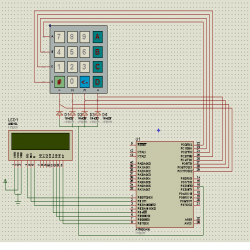
Code:
#include<avr/io.h>
#include<util/delay.h>
#include<lcd.h>
#include<keypad.h>
void function()
{
LCDclr();
LCDGotoXY(0,0);
LCDdisplay("ENTER PASSWORD");
LCDGotoXY(1,0);
}
int i=0,j=0;
char k=0,arr[16]="NUL",pass[16]="1234";
void main()
{
DDRA=1;
PORTA=0;
LCDinit();
LCDcursorOFF();
while(1)
{
if(PINA&1)
{
LCDdisplay("SAFE OPEN");
LCDGotoXY(1,0);
}
else
function();
while(i<15000)
{
if(!k)
i++;
else
{
if(k!='#' && k!='E')
{
arr[j++]=k;
if(PINA&1)
LCDsendChar(k);
else
LCDsendChar('*');
}
else if(k=='E' && j!=0)
{
j--;
LCDcursorLeft(1);
LCDsendChar(' ');
LCDcursorLeft(1);
}
else if(k!='E')
{
if(!strcmp(arr,"NUL"))
{
PORTA=0;
LCDclr();
LCDdisplay("SAFE LOCKED");
_delay_ms(1000);
function();
}
else
{
arr[j]='\0';
j=0;
LCDclr();
if(PINA&1)
{
if(strlen(arr)>=4)
{
strcpy(pass,arr);
PORTA=0;//lock the safe
LCDclr();
LCDdisplay("SAFE LOCKED");
_delay_ms(1000);
function();
}
else
{
LCDdisplay("ERROR");
LCDGotoXY(1,0);
LCDdisplay("WEAK PASSWORD");
_delay_ms(1000);
LCDclr();
LCDdisplay("SAFE OPEN");
LCDGotoXY(1,0);
}
}
else
{
if(!strcmp(arr,pass))
{
LCDdisplay("SAFE OPEN");
LCDGotoXY(1,0);
PORTA=1;//open the safe
}
else
{
LCDdisplay("ERROR");
LCDGotoXY(1,0);
LCDdisplay("WRONG PASSWORD");
_delay_ms(1000);
function();
}
}
strcpy(arr,"NUL");
}
}
i=0;
}
k=Read_Keypad();
}
LCDclr();
DDRC=0x0f;
PORTC=0x00;
SREG|=(1<<7); //initializes the global interrupt i.e. sei();
DDRD&=~(1<<2); //declares INT0 as input pin
PORTD|=(1<<2); //pull-up enabled
GICR|=(1<<INT0); //enables INT0
GIFR|=(1<<INTF0); //enables INT0 flag for ISR recognition
MCUCR|=(1<<SM0)|(1<<SM1)|(1<<SE); //sleep mode enabled
MCUCR&=~(1<<SE); //sleep mode disabled
}
}
ISR(INT0_vect)
{
k=Read_Keypad();
GICR&=~(1<<INT0);
GIFR&=~(1<<INTF0);
i=0;j=0;strcpy(arr,"NUL");
}
Không có nhận xét nào:
Đăng nhận xét